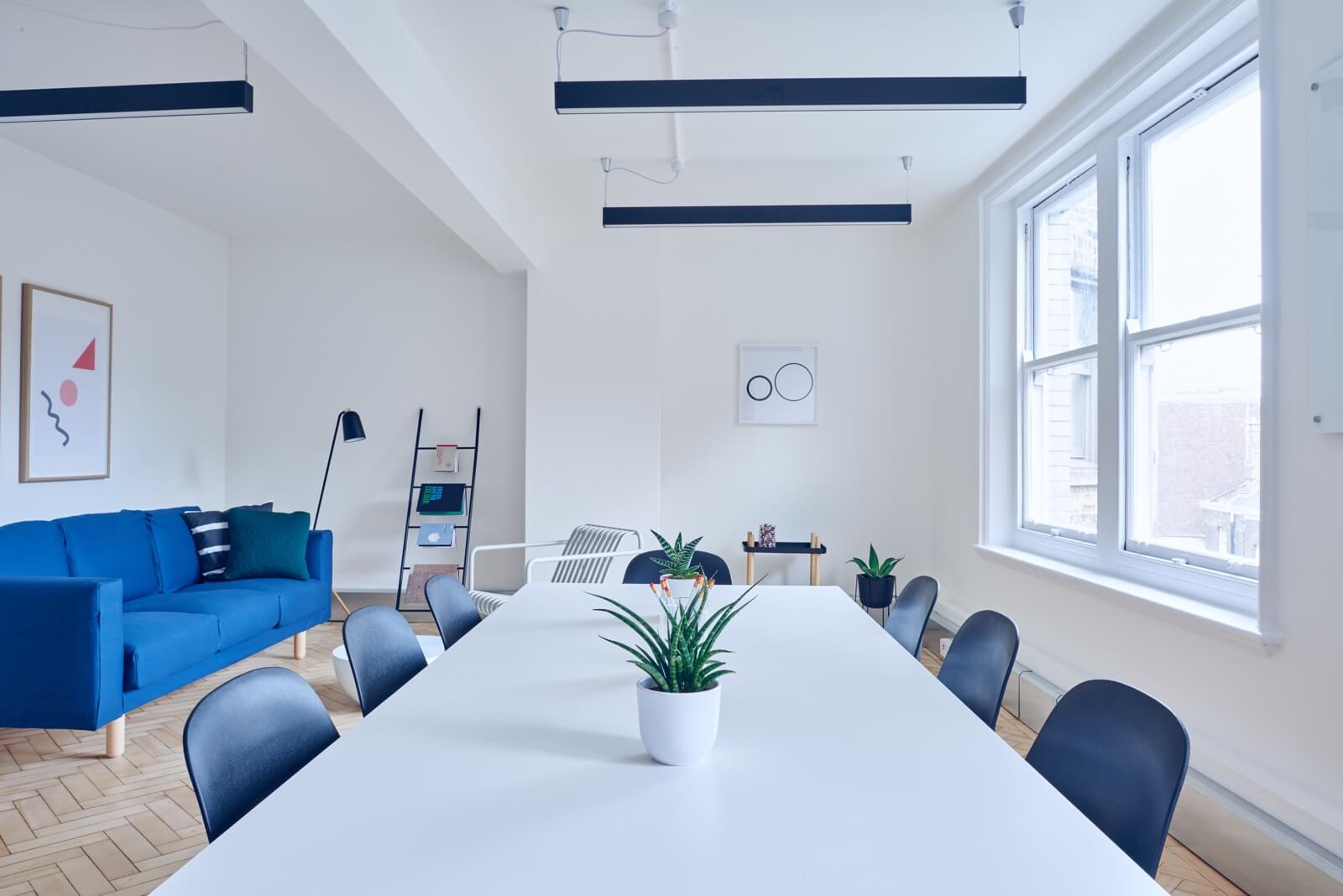
Tradematic Support Center
Guides, articles, videos and links for Tradematic users and developers.
Signal execution inside the bar (by touch/by break)
137185ARTICLE CODE EDITOR BREAKOUT BREAK TOUCH INSIDE BAR BUYATSTOP SELLATLIMIT RUN EVERYWhat are the types of signal execution in Tradematic?
In Tradematic Trader there are two types of signal execution – by the closing price of bar and inside the bar.
If signal execution is set as “by the closing price of bar”, trading signal will be executed after closing of the bar. We highly recommend all new users to use this variant.
If signal execution is set as “inside the bar”, trading signal will be executed the exact moment, when it was generated. This gives a higher speed of reacting to market changes, but it also increases chances of executing a false signal. In addition, closing price of bar often tend to be more profitable, than the price at the moment, when signal was generated.
We do not recommend to use execution inside the bar unless you become a skilled user and know how to use it properly.
Example of strategy that trades by the closing price of bar
As an example we can create a strategy that uses simple moving averages: we open position when High is above middle SMA with period 18, and we close position when Low is below the middle SMA with period 18.
Here is the pre class='prettyprint' of this strategy:
using System; using System.Collections.Generic; using System.Text; using System.Drawing; using TradeMatic; using TradeMatic.Indicators; namespace ScriptNamespace { class MyScript : Script { private StrategyParameter parameter0; public MyScript() { parameter0 = CreateParameter("SMA Period", 18, 0, 1000, 1); } public override void Execute() { // Display indicators on the chart PlotSeries(PricePane, SMA.Series(Close, parameter0.ValueInt), Color.Red, LineStyle.Solid, 1); // Initialization // Main cycle for (int bar = 18; bar < Symbol.Count; bar++) { if (MarketPosition == 1) { // Closing long position if ((Low[bar] < SMA.Value(bar, Close, parameter0.ValueInt))) { SellAtClose(bar, LastPosition, ""); } } else { // Opening long position if ((High[bar] > SMA.Value(bar, Close, parameter0.ValueInt))) { BuyAtClose(bar, ""); } } } } } }Now we need to save the strategy and backtest it. Let’s look at the chart with prices:
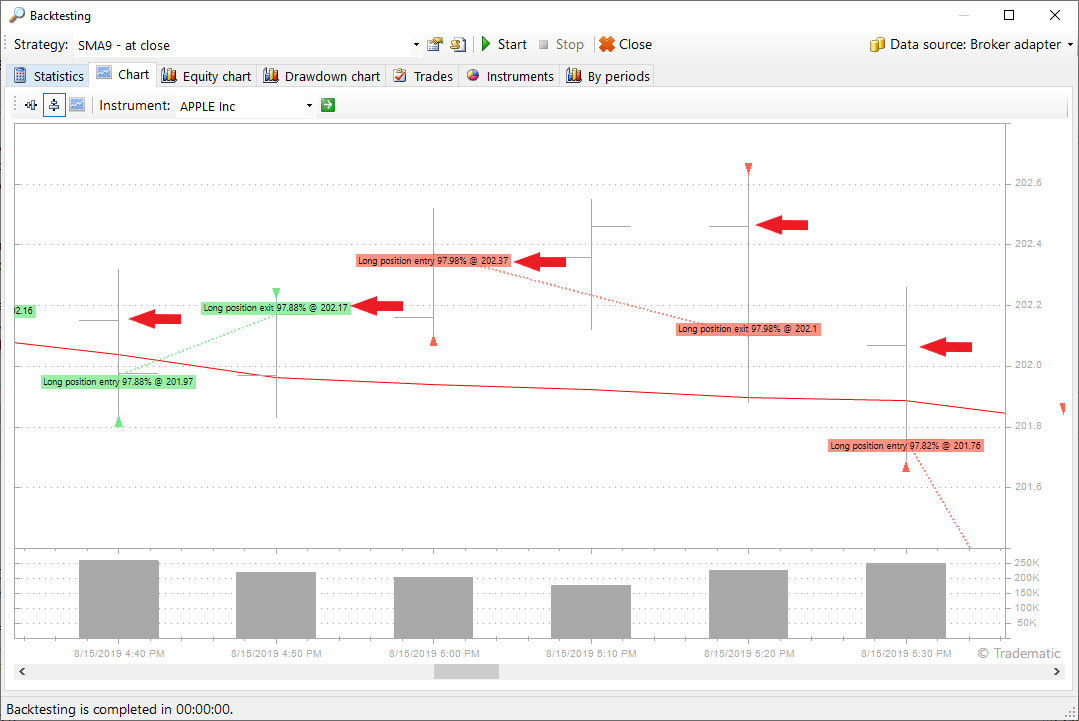
Entries prices are marked by big red arrows. We see that in most cases the strategy has opened position by a much higher price (by closing price of bar), comparing to price which was at the moment of SMA crossover.
Similarly, strategy has closed position by a lower price.
How to change signal execution type for strategy from execution by closing price of bar to execution inside the bar?
Let’s make such changes and see the results. To do this, we need to change a little bit strategy pre class='prettyprint' in Code Editor.
We implement changes in several steps.
1. Change all Close prices to High or Low.
Why we need to do this? In case of signal execution inside the bar, the strategy is executed several times (“Execute every” parameter) inside the main timeframe of strategy (“Timeframe” parameter).
Thus, each time strategy is executed, the last (current) bar Close price is the price of the last trade by the moment of strategy execution. So the last (current) bar Close price can differ from the final closing price of this bar.
This can lead to the following problem. Inside the bar the Close price is above SMA(18) during a few moments, so the signal is executed and position is opened. But the final closing price is below SMA(18), so the strategy counts that position is not opened. So changing Close to High or Low is highly recommended. In this example we don’t use Close prices, so we don’t change it.
2. Change position opening and closing functions from using closing prices to prices of crossover.
We change BuyAtClose to BuyAtStop, and SellAtClose to SellAtLimit. In these functions we need to set prices for execution.
These functions consist of 2 parts – Position and Price.
1. Position:
Buy — open long position
Sell — close long position
Short — open short position
Cover — close short position
2. Price:
AtClose — execute by the closing price of bar
AtMarket — execute by the opening price of bar
AtLimit — execute by set price, if it is greater than or equal to Low price of bar
AtStop — execute by set price, if it is less than or equal to High price of bar
For example, BuyAtStop function consists of “Buy” and “AtStop”.
In our example this is SMA.Value().
The final pre class='prettyprint' of our strategy looks the following way:
using System; using System.Collections.Generic; using System.Text; using System.Drawing; using TradeMatic; using TradeMatic.Indicators; namespace ScriptNamespace { class MyScript : Script { private StrategyParameter parameter0; public MyScript() { parameter0 = CreateParameter("SMA Period", 18, 0, 1000, 1); } public override void Execute() { // Display indicators on the chart PlotSeries(PricePane, SMA.Series(Close, parameter0.ValueInt), Color.Red, LineStyle.Solid, 1); // Initialization // Main cycle for (int bar = 18; bar < Symbol.Count; bar++) { if (MarketPosition == 1) { // Closing long position if ((Low[bar] < SMA.Value(bar, Close, parameter0.ValueInt))) { SellAtStop(bar, SMA.Value(bar, Close, parameter0.ValueInt), LastPosition, ""); } } else { // Opening long position if ((High[bar] > SMA.Value(bar, Close, parameter0.ValueInt))) { BuyAtLimit(bar, SMA.Value(bar, Close, parameter0.ValueInt), ""); } } } } } }
Let’s compare the results of trades:
Strategy that trades by closing price of bar:
Strategy that trades by touch inside the bar:
As we can see, strategy that trades by touch showed better results.
What happens when signal for position opening arrives several times inside the same bar?
In Tradematic Trader there is a prevention from executing signals of the same direction within the same bar. So if several such signals arrive, only the first one will be executed.
How to launch a strategy with execution inside the bar?
After implementing the changes and testing it:
1) Unblock “Execute every” parameter. To do this, open Tradematic Trader settings (“Instruments” menu button -> Settings), then choose “Strategies” tab and tick “Enable signal execution inside the bar”.
2) Open strategy properties (right-click the strategy -> Strategy properties). Choose “Basic parameters” tab and set the “Execute every” parameter. This parameter must be less than or equal to strategy main timeframe. If “Execute every” parameter is equal to “Timeframe” parameter, this means that strategy trades by closing price of bar.
How to use risk management (stop-loss, take-profit) inside the bar?
Standard stop-loss and take-profit (which you can find in Visual strategy builder) use the closing price of bar, so they are not for such case.
But we can add risk management by setting signal execution price in SellAtLimit and SellAtStop functions:
if (MarketPosition == 1) { // Closing long position // Take-profit – if price grows by 5% SellAtLimit(bar,LastPosition.EntryPrice*1.05, LastPosition, "TP (LX)"); // Stop-loss – if price falls by 3% SellAtStop(bar,LastPosition.EntryPrice*0.97, LastPosition, "SL (LX)"); // other conditions for closing long position ...We have added take-profit for price increase by 5%, and stop-loss for price decrease by 3%. This pre class='prettyprint' should be added to the block of closing position.